[Kate, one of our developers at SoftArtisans, was pulled onto a project to do consulting work. Below, Kate discusses her work and how she learned to handle inherited code. Read about the lessons she’s learned after being gifted a whole new project of code.]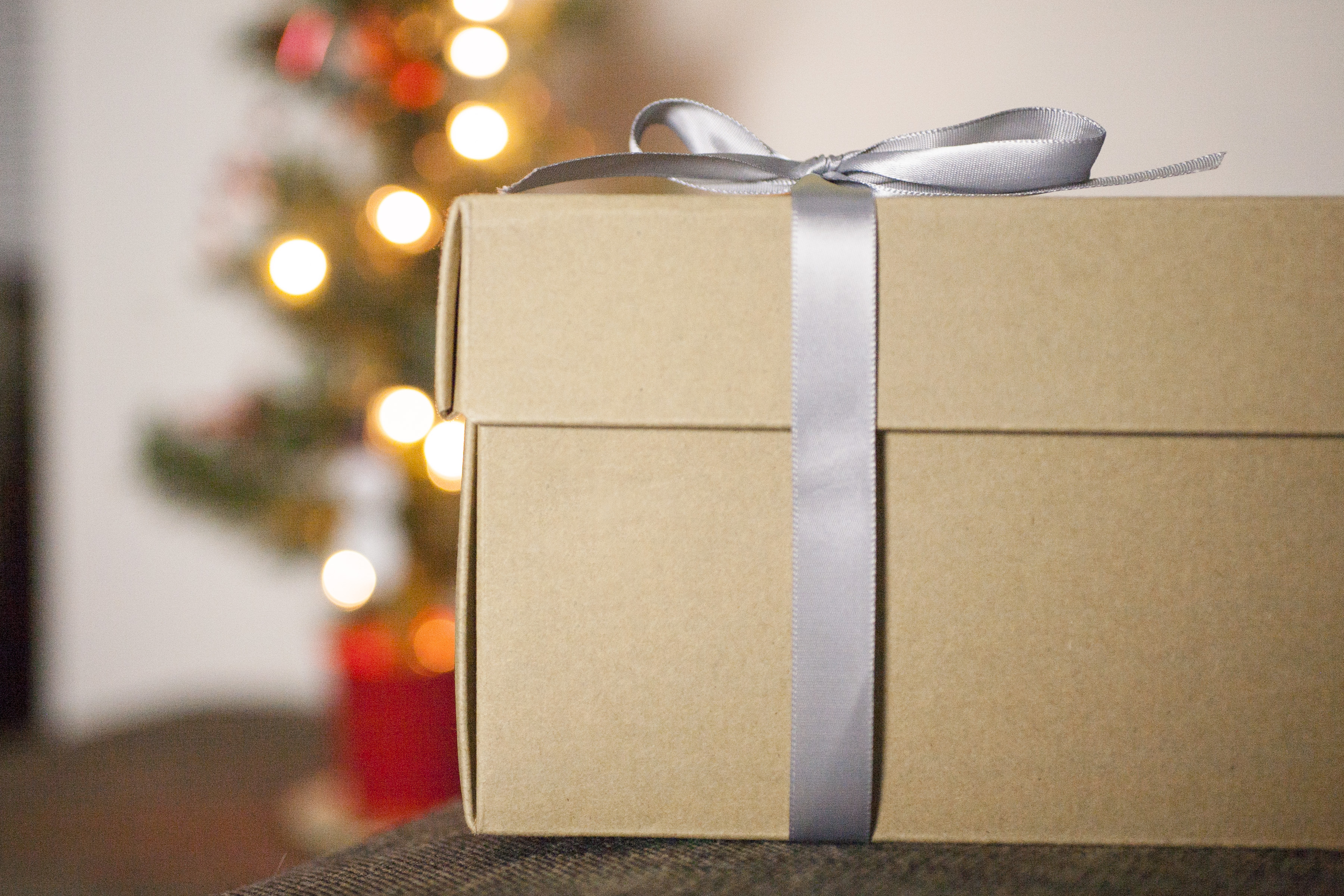
Recently, I inherited the code for a pretty large project, and anyone who knew the application wasn’t around when I took it over. Learning a brand new application is a daunting task – doubled if you have to learn it without any outside resources. As I tried to familiarize myself with the application, I picked up a few tricks to handling inherited code. These are a few of the things I learned along the way that will hopefully save you hours on your next undertaking.
1. Resist temptation
It can be really hard to ignore glaring implementation flaws when you look at code for the first time. Unfortunately, there are often reasons that the junky code exists in the first place. It might not be the best solution, but if it works, just leave it. Until you fully understand what each piece of code does, you might break something unintentionally by trying to fix it. Now you’ve lost time and still don’t understand the code any better. Unless it’s necessary for your current scope of work, make a tech debt note about why it’s bad code, and back away slowly.
2. Check the docs
I learn by immediately messing around in the code and doing as much damage as possible before rolling back. This isn’t really great, but hands-on learning is so natural for most technical things that we forget about reading. You. Yes, you. You are a developer so reading seems really unnecessary. Unfortunately, we still haven’t found a way to automate it, so you’ll have to do the reading yourself. Look at any documentation that might be around. It’ll suck. It won’t make a bit of sense. Then in 6 months, you’ll look at a weird method and remember that the docs said why it was like that. And you’ll think to yourself, “Ah, well, Old Fellow, looks like that reading did more than a mite bit of good!” and you’ll laugh and light up a cigar with your adoring fans swooning and giggling. Unless, you know, you’re a normal person and not a caricature of an old famous British man. Which brings me to 3…
3. Be a caricature of an old famous British man. No. Kidding. Don’t do this. (But if you do this, let’s be friends.)
3. Check the change log
It’s useful to see what might have been done recently, what kinds of issues have been encountered, and where those fixes lie. If there’s bug tracking, change logs, general progress docs, find them all. You don’t have to memorize every issue, but go over some known problems and keep yourself from making the same mistakes.
4. Be a user
It’s a lot easier to understand the code if you take some time to use the actual application. Use it like a user would, ask users (or consult the people who already did), find out why this is the way it is. There might be a good reason that all the buttons are yellow and labeled “Do not click.” It might help the users’ workflow. Make sure you know, because ultimately if the people who need this hate what you’ve done, someone else will be inheriting this code from you.
5. Just ask
When you inherit code blind, it’s awful. I mean, of course, the last ten people to look at this have all left the company/country/planet, and you’re all alone and in the dark. However, it doesn’t hurt to ask around and find out if anyone else knows what’s up. Alternately, if you have someone who knows the code, don’t get stuck in “Am I seriously doing this wrong?” hell, and just ask the question. 9 times out of 10, the code is confusing because it’s confusing code.
6. Look before you implement
It’s great when you can finally work on the code you’ve inherited. Continue reading 10 Tips for Handling Inherited Code →